When creating an instance of SpotfireDocument, you can control pretty much any aspect of the Spotfire instance with it.
However, this instance can only be done in the current document and cannot be shared to other iframes if they resides on a different domain.
This is what happens for example in the SWAPP. When we load the result page of a protocol, this is done in an iframe. If the iframe is on the same domain, we can easily pass it the instance of the SpotfireDocument
. It can then manipulate the document easily. But if the frame is on a different domain, this can cannot be done. In this case, the only way to communicate between the two frame is though the Message API.
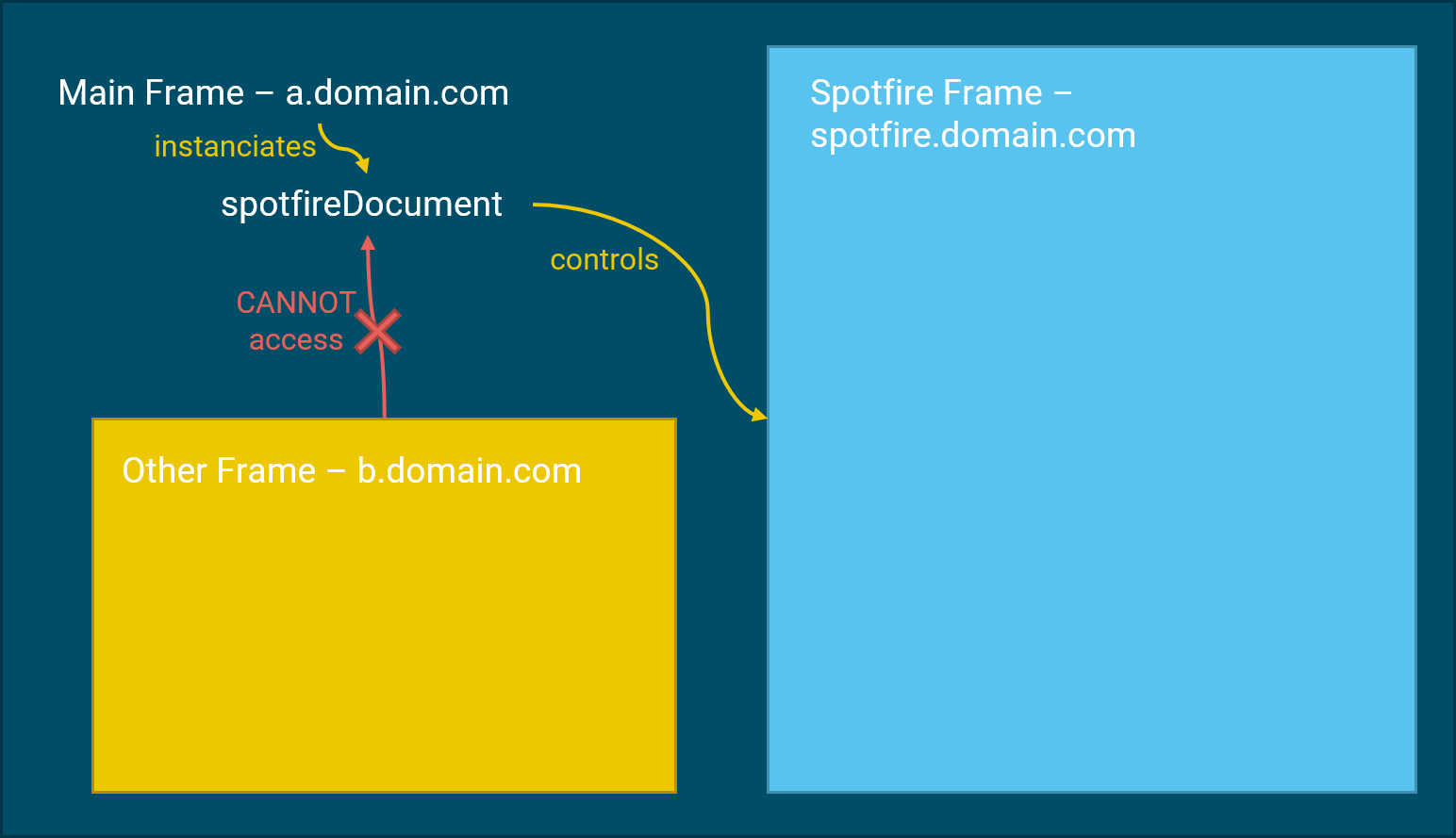
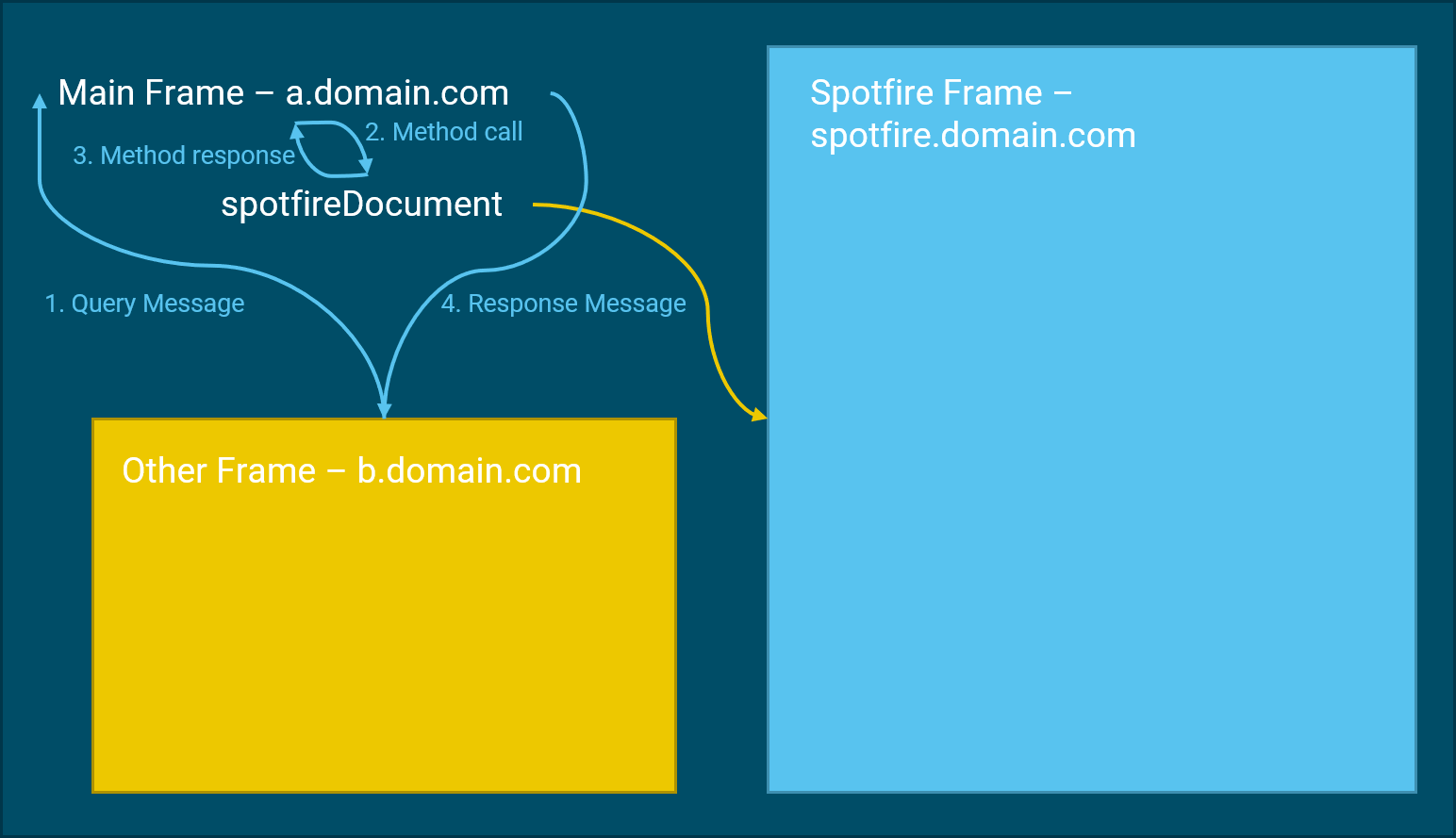
To simplify the implementation of the cross-frame communication, we provide two classes: SpotfireMessageHandler and SpotfireDocumentProxy.
The SpotfireMessageHandler
class must be instanciated on the frame containing the SpotfireDocument
instance.
It takes as argument the SpotfireDocument
instance and optionally the domain of the frame we target.
If we do not set the target frame domain, the wildward "*" will be used. For security reasons, it is strongly recommended to set the domain.
In our case, that would be:
// On Main frame
const spotfireDocument = new SpotfireDocument({
serverUrl: 'https://spotfire.domain.com',
documentPath: '/Discngine/Client Automation/empty'
})
const spotfireMessageHandler = new SpotfireMessageHandler(spotfireDocument, 'https://b.domain.com')
On the other frame, located in b.domain.com, we instanciate the SpotfireDocumentProxy
class.
It takes the window object of the target frame (in our case window.parent
) and similarly the target frame domain which is optional but strongly recommended.
Once done, we can then use it to call any method of the SpotfireDocument instance.
Because the message API is asynchronous, all methods of the SpotfireDocumentProxy
are asynchronous as well, even those which are synchronous on the SpotfireDocument like createWhereClauseForColumnAndValues.
const spotfireDocumentProxy = new SpotfireDocumentProxy(window.parent, 'https://a.domain.com');
// Then we can use any method to retrieve data.
const test = async () => {
const activeDataTable = await spotfireDocumentProxy.getActiveDataTableAsync()
console.log(activeDataTable);
const properties = await spotfireDocumentProxy.getPropertiesAsync();
console.log(properties)
}
test()